1 #include 2 #include 3 using namespace std; 4 5 enum error{overflow,underflow,success}; 6 const int maxlen=100; 7 8 class queue{ 9 public:10 queue();//初始化11 bool empty()const;//判断为空12 bool full()const;//判断为满13 int get_front(int &x)const;//取队头元素14 error append(const int x);//入队15 error serve();//出队16 private:17 int count;//队列中元素计数18 int rear,front;//队头,队尾19 int data[maxlen];//存储队列中的数据20 };21 queue::queue(){ //初始化22 count=0;23 rear=front=0;24 }25 26 bool queue::empty()const{ //判断为空27 if(count==0)return true;28 return false;29 }30 31 bool queue::full()const{ //判断为满32 if(count==maxlen-1)return true;33 return false;34 }35 36 int queue::get_front(int &x)const{ //取队头元素37 if(empty())return underflow;38 x=data[(front+1)%maxlen];39 return success;40 }41 error queue::append(const int x){ //入队42 if(full())return overflow;43 rear=(rear+1)%maxlen;44 data[rear]=x;45 count++;46 return success;47 }48 49 error queue::serve(){ //出队50 if(empty())return underflow;51 front=(front+1)%maxlen;52 count--;53 return success;54 }55 56 int main(){57 queue q;58 int n;59 cout<<"please input 杨辉三角要打印的行数:";60 cin>>n;61 int s1,s2;62 for(int i=1;i <<" ";63 cout<<1<
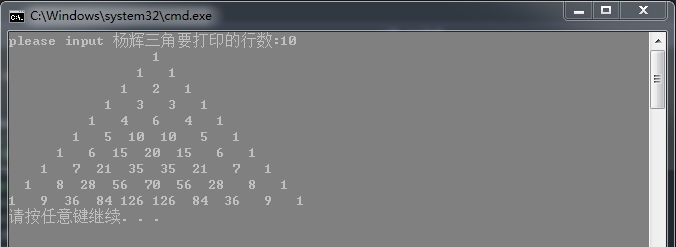